This document will guide you on how to correctly integrate Zip with PowerBoard.
Before you begin
When sending requests to PowerBoard’s API, you must provide either a Public Key and/or Secret Key depending on your use case.
API keys are generated via PowerBoard’s Merchant Dashboard, in three easy steps:
- Login to the PowerBoard Merchant Dashboard.
- Click on the ‘My Company’ link and proceed to the ‘API and Settings’ heading.
- On screen, you’ll see both Public and Secret Key required to send requests to PowerBoard’s API.
Third-Party Requirements
- Zip merchant account connected (sandbox mode) via PowerBoard Dashboard.
- Zip test customer account
Your integration will utilise the following components:
- PowerBoard Client-SDK ('Widget')
- PowerBoard REST API
Integration Steps
Step 1) Create and initialise the PowerBoard Widget
Embed PowerBoard's Client-SDK on your page.
Create a button container for the PowerBoard widget.
<script src="https://widget.preproduction.powerboard.commbank.com.au/sdk/latest/widget.umd.min.js"></script>
<button type="button" id="button-zip">Click to launch ZipPay!</button>
Step 2) Configure Client-SDK
Configure the following parameters within the cba.ZipmoneyCheckoutButton class:
- setBackdropDescription
- Description which can be shown in the checkout page.
- setBackdropTitle
- Title which can be shown in overlay of the checkout page.
- setEnv('preproduction_cba')
- Sets Client-SDK ('Widget') to PowerBoard Pre-Production (test) environment.
<script src="https://widget.preproduction.powerboard.commbank.com.au/sdk/latest/widget.umd.min.js"></script>
<button type="button" id="button-zip">Click to launch ZipPay!</button>
<script>
var button = new cba.ZipmoneyCheckoutButton('#button-zip', 'YOUR_PUBLIC_KEY', 'GATEWAY_ID');
button.setBackdropDescription('PowerBoard Zip Demo');
button.setBackdropTitle('Zip Transaction');
button.setEnv('preproduction_cba');
</script>
Step 3) Event Handling
PowerBoard allows for event handling through the Client-SDK, please see below valid event types and examples.
Event Name | Example |
---|---|
click | button.on('click', function (data) { console.log('click'); }); |
popupRedirect | button.on('popupRedirect', function (data) { console.log('popupRedirect'); }); |
accepted | button.on('accepted', function (data) { console.log('accepted'); }); |
finish | button.on('finish', function (data) { console.log('finish event', data); }); |
error | button.on('error', function (data) { console.log('error'); }); |
close | button.on('close', function(data) { console.log('close'); }); |
<script src="https://widget.preproduction.powerboard.commbank.com.au/sdk/latest/widget.umd.min.js"></script>
<button type="button" id="button-zip">Click to launch ZipPay!</button>
<script>
var button = new cba.ZipmoneyCheckoutButton('#button-zip', 'YOUR_PUBLIC_KEY', 'GATEWAY_ID');
button.setBackdropDescription('PowerBoard Zip Demo');
button.setBackdropTitle('Zip Transaction');
button.setEnv('preproduction_cba');
button.on('click', function (data) {
console.log('click');
});
button.on('finish', function (data) {
console.log('finish event', data);
});
button.on('popupRedirect', function (data) {
console.log('popupRedirect');
});
button.on('accepted', function (data) {
console.log('accepted');
});
button.on('error', function (data) {
console.log('error');
});
button.on('close', function (data) {
console.log('close');
});
</script>
Step 4) Construct meta information for the checkout page
Use .setMeta method to provide information about your Zip transaction with the following parameters:
Field | Required | Type | Description |
---|---|---|---|
first_name | Y | string | Customer first name |
last_name | Y | string | Customer last name |
tokenize | N | string | Tokenize transaction flag |
Y | string | Customer email address | |
gender | N | string | Customer gender |
charge.amount | Y | string | Total amount for the transaction |
charge.currency | Y | string | Always set to 'AUD' |
shipping_address.first_name | Y | string | Shipping first name |
shipping_address.last_name | Y | string | Shipping last name |
shipping_address.line1 | Y | string | Shipping Address, line 1 |
shipping_address.line2 | Y | string | Shipping Address, line 2 |
shipping_address.country | Y | string | Shipping Address, country |
shipping_address.postcode | Y | string | Shipping Address, postcode |
shipping_address.city | Y | string | Shipping Address, city |
shipping_address.state | Y | string | Shipping Address, state |
billing_address.first_name | Y | string | Billing first name |
billing_address.last_name | Y | string | Billing last name |
billing_address.line1 | Y | string | Billing Address, line 1 |
billing_address.line2 | Y | string | Billing Address, line 2 |
billing_address.country | Y | string | Billing Address, country |
billing_address.postcode | Y | string | Billing Address, postcode |
billing_address.city | Y | string | Billing Address, city |
billing_address.state | Y | string | Billing Address, state |
items.name | Y | string | Name of item within the charge |
items.amount | Y | string | Amount of item within the charge |
items.quantity | Y | string | Quantity of items within the charge |
items.reference | Y | string | Reference for items within the charge |
<script src="https://widget.preproduction.powerboard.commbank.com.au/sdk/latest/widget.umd.js"></script>
<button type="button" id="button-zip">Click to launch ZipPay!</button>
<script>
var button = new cba.ZipmoneyCheckoutButton('#button-zip', 'YOUR_PUBLIC_KEY', 'GATEWAY_ID');
button.setBackdropDescription('PowerBoard Zip Demo');
button.setBackdropTitle('Zip Transaction');
button.setEnv('preproduction_cba');
button.on('click', function (data) {
console.log('click');
});
button.on('popupRedirect', function (data) {
console.log('popupRedirect');
});
button.on('accepted', function (data) {
console.log('accepted');
});
button.on('error', function (data) {
console.log('error');
});
button.on('close', function (data) {
console.log('close');
});
button.setMeta({
"first_name": "John",
"tokenize": false,
"last_name": "Citizen",
"email": "[email protected]",
"gender": "male",
"charge": {
"amount": "100.00",
"currency": "AUD",
"shipping_address": {
"first_name": "John",
"last_name": "Citizen",
"line1": "123456 Test Street",
"line2": "Unit 1",
"country": "AU",
"postcode": "2000",
"city": "Sydney",
"state": "NSW"
},
"billing_address": {
"first_name": "John",
"last_name": "Citizen",
"line1": "123 Test Street",
"line2": "Unit 123",
"country": "AU",
"postcode": "2000",
"city": "Sydney",
"state": "NSW"
},
"items": [{
"name": "Shoes 1",
"amount": "50.00",
"quantity": 1,
"reference": "My_Example_Merchant_Reference_01"
}, {
"name": "Shoes 2",
"amount": "50.00",
"quantity": 1,
"reference": "My_Example_Merchant_Reference_02"
}]
}
});
</script>
Step 5) Send Payment Token to PowerBoard's API
The PowerBoard Client-SDK will trigger the 'finish' event once the end-user has completed the Zip workflow (see below).
Field | Description |
---|---|
payment_source_token | PowerBoard payment source token used in Charge API request. |
checkout_email | Payer's email address listed on Zip account. |
checkout_holder | Payer's name listed on Zip account. |
gateway_type | PowerBoard gateway service type. |
{
"payment_source_token": "POWERBOARD_PAYMENT_SOURCE_TOKEN",
"checkout_email": "[email protected]",
"checkout_holder": "John Citizen",
"gateway_type": "Zipmoney"
}
Use the 'Payment_Source_Token
' from the 'finish' event within your Charge API request.
API Endpoint | https://api.preproduction.powerboard.commbank.com.au/v1/charges |
---|---|
HTTP Method | POST |
Headers | x-user-secret-key- POWERBOARD_SECRET_KEY - This is your PowerBoard API Secret Key. Content-Type - application/json - Type will always be application/json. |
Request Parameters | amount - string - Total amount for the transaction. transactions.currency - string - Always set to 'AUD'. token - string - payment_source_token returned from the 'finish' event. Example: "payment_source": "c2a69078-110c-4081-a927-167d9d1b2f04" |
Charge Request with Zip Payment Source Token
PowerBoard will respond with a '201 Created', respectively. This response should be stored against your database or relevant payments ecosystem.
{
"amount": "10.00",
"currency": "AUD",
"token": "251985e6-a368-4168-8e1a-bfb30c3e4bab"
}
{
"_id": "63ec23f1a412be2c507ced2a",
"transfer": {
"items": []
},
"schedule": {
"stopped": false
},
"statistics": {
"total_refunded_amount": 0,
"full_refund": false,
"need_sync": true
},
"customer": {
"payment_source": {
"type": "checkout",
"gateway_id": "63cf467933020e1cd63006d1",
"gateway_name": "Zipmoney",
"gateway_type": "Zipmoney",
"ref_token": "type:Y2hlY2tvdXRfaWQ=.token:Y29fMVpQQ0xIaVBrVmhXMlM2c1FDa3VG",
"address_line1": "123 Test Street",
"address_line2": "Unit 123",
"address_city": "Sydney",
"address_postcode": "2000",
"address_state": "NSW",
"address_country": "AU"
},
"first_name": "John",
"last_name": "Citizen",
"email": "[email protected]"
},
"shipping": {
"address_line1": "123 Test Street",
"address_line2": "Unit 123",
"address_country": "AU",
"address_postcode": "2000",
"address_city": "Sydney",
"address_state": "NSW"
},
"type": "financial",
"status": "complete",
"capture": true,
"authorization": false,
"archived": false,
"one_off": true,
"_source_ip_address": "0.0.0.0",
"amount": 0.4,
"currency": "AUD",
"items": [],
"company_id": "63cf32a154a870183bf2398a",
"amount_surcharge": null,
"amount_original": null,
"updated_at": "2023-02-15T00:14:43.454Z",
"created_at": "2023-02-15T00:14:41.968Z",
"__v": 0,
"external_id": "ch_gyeg1v245884vek"
}
Additionally, you will see the charge appear in the PowerBoard Merchant Dashboard.
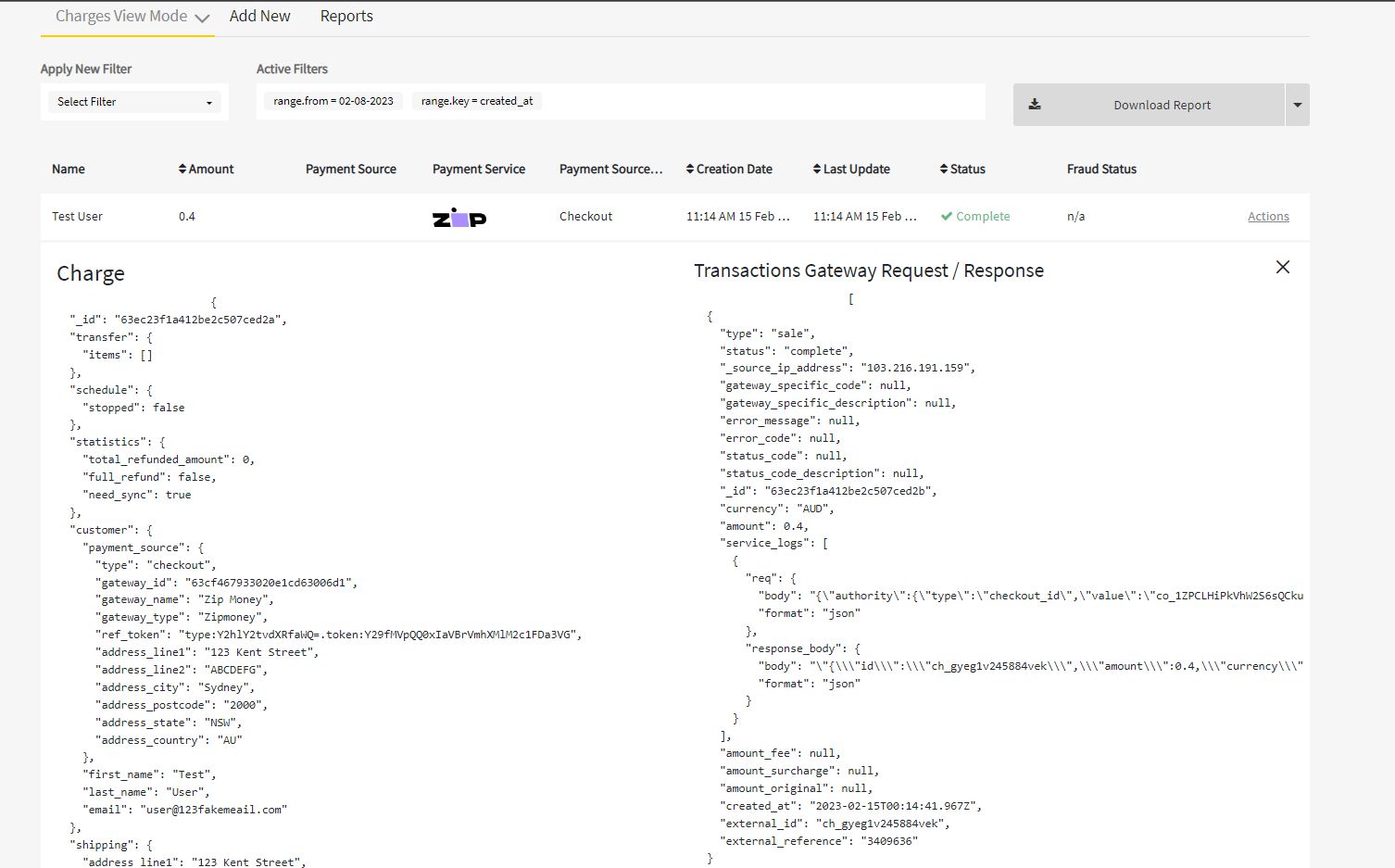