This guide will provide you with instructions on how-to initialise & customise your PowerBoard Widget integration.
What is the Hosted Client SDK?
PowerBoard offers an extensively customisable client sdk that accepts all available payment methods, supports input validation, and built-in 3DS 2.0 capability.
- It is a solution for collecting and handling payment sources in a secure way.
- With the client SDK you can create a payment form widget as an independent part or use it directly inside your payment form.
- The SDK supports a number of methods for customising the widget to your websites needs (styling, form fields, etc)
Getting started with the Client SDK
To work with the widget you will need public_key or access_token
Also you will need a gateway id from your connected services in PowerBoard.
Environments
<script src="https://widget.preproduction.powerboard.commbank.com.au/sdk/latest/widget.umd.min.js"></script>
<script>
var widget = new cba.HtmlWidget('#widget', 'PUBLIC_KEY', 'GATEWAY_ID');
widget.setEnv("preproduction_cba");
widget.load();
</script>
<div id="widget"></div>
<script src="https://widget.powerboard.commbank.com.au/sdk/latest/widget.umd.min.js"></script>
<script>
var widget = new cba.HtmlWidget('#widget', 'PUBLIC_KEY', 'GATEWAY_ID');
widget.setEnv("production_cba");
widget.load();
</script>
<div id="widget"></div>
Widget Customisation
Please note that the steps and code samples are generalised, so you may need to adjust them to fit your application.
Widget customisation demo tool
This interactive app will demonstrate an example of how you can customise the PowerBoard Widget to your website requirements.
widget.setStyles({
background_color: '#FFFFFF',
border_color: '#000000',
text_color: '#000000',
button_color: ,
font_size: '14px',
font_family: 'Trebuchet MS'
});
widget.setTexts({
title: 'Your Card Details',
title_description: '* indicates required field',
submit_button:: ,
submit_button_processing::
});
Widget Classes
setFormFields
Description
Set list with widget form field, which will be shown in form. Also, you can set the required validation for these fields.
widget.setFormFields(['phone',
'email',
'first_name*',
'last_name',
'phone2',
'address_line1',
'address_line1',
'address_line2',
'address_state',
'address_country',
'address_city',
'address_postcode',
'address_company'
]);
Configurable Parameters
Parameter | Type |
---|---|
card_name | string |
expire_month | string |
expire_year | string |
card_ccv | string |
first_name | string |
last_name | string |
string | |
phone | string |
phone2 | string |
address_line1 | string |
address_line2 | string |
address_state | string |
address_city | string |
address_postcode | string |
address_company | string |
Preview
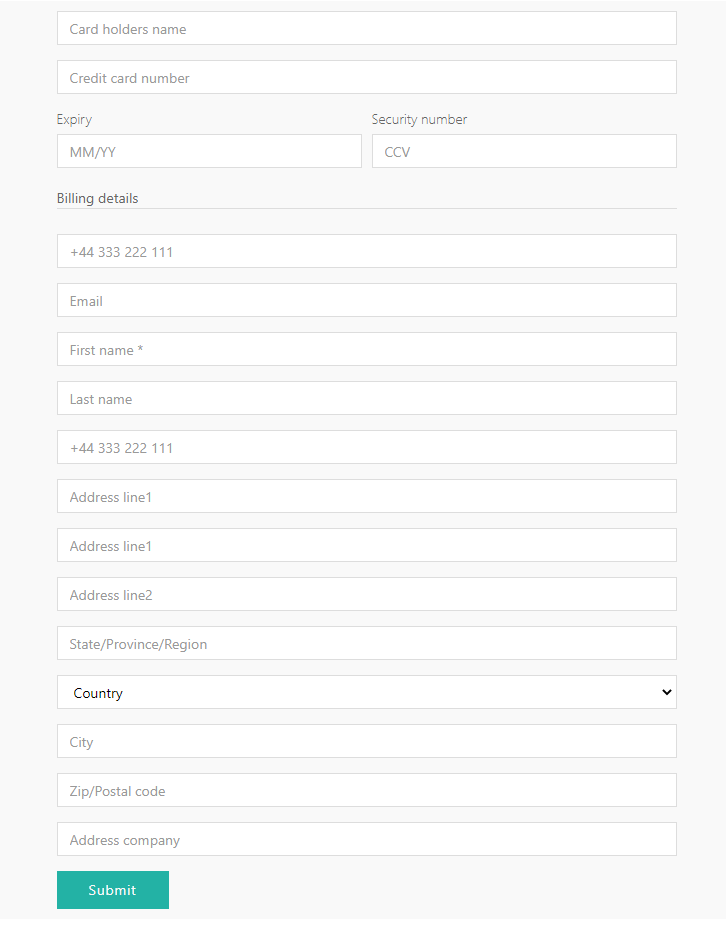
setFormElements
Description
Allows merchants to set and configure Widget Form elements, including:
- visibility
- required
- label
- placeholder
- value
widget.setFormElements([
{
field: 'card_name*',
placeholder: 'Input your card holder name...',
label: 'Card Holder Name',
value: 'Houston',
},
{
field: 'email',
placeholder: 'Input your email, like [email protected]',
label: 'Email for the receipt',
value: '[email protected]',
},
])
Configurable Parameters
Parameter | Type |
---|---|
card_name | string |
expire_month | string |
expire_year | string |
card_ccv | string |
first_name | string |
last_name | string |
string | |
phone | string |
phone2 | string |
address_line1 | string |
address_line2 | string |
address_state | string |
address_city | string |
address_postcode | string |
address_company | string |
Preview
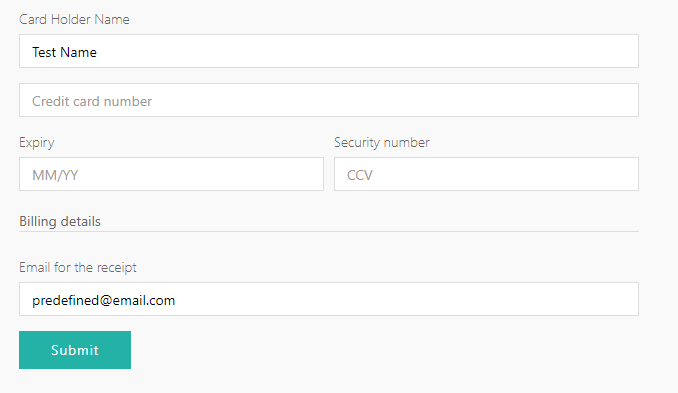
setStyles
Description
Allows to set and configure styling for the Widget
widget.setStyles({
background_color: 'rgb(0, 0, 0)',
border_color: 'yellow',
text_color: '#FFFFAA',
button_color: 'rgba(255, 255, 255, 0.9)',
font_size: '20px',
font_family: 'fantasy'
});
Configurable Parameters
Parameter | Type |
---|---|
background_color | string |
text_color | string |
border_color | string |
button_color | string |
error_color | string |
success_color | string |
font_size | string |
font_family | string |
setTexts
Description
Method to set different texts within the widget.
- visibility
- required
- label
- placeholder
- value
widget.setTexts({
title: 'Your card',
finish_text: 'Payment resource was successfully accepted',
title_description: '* indicates required field',
submit_button: 'Save',
submit_button_processing: 'Load...',
});
Configurable Parameter
Parameter | Type |
---|---|
[title] | string |
[title_h1] | string |
[title_h2] | string |
[title_h3] | string |
[title_h4] | string |
[title_h5] | string |
[title_h5] | string |
[title_h6] | string |
[finish_text] | string |
[title_description] | string |
[submit_button] | string |
[submit_button_processing] | string |
Preview
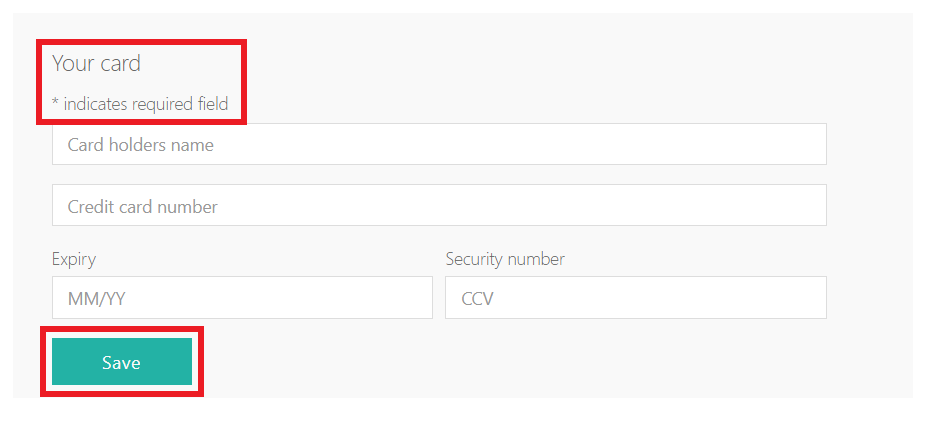
setElementStyle
Description
Method for setting customisation of the PowerBoard Widget elements and relevant states.
Element states are as follows:
- error
- focus
- hover
widget.setElementStyle('input', {
border: 'green solid 1px'
});
widget.setElementStyle('input', 'focus', {
border: 'blue solid 1px'
});
widget.setElementStyle('input', 'error', {
border: 'red solid 1px'
});
widget.setElementStyle('input', 'hover', {
border: 'blue solid 1px'
});
Configurable Parameter
Parameter | Type |
---|---|
[title] | string |
[title_h1] | string |
[title_h2] | string |
[title_h3] | string |
[title_h4] | string |
[title_h5] | string |
[title_h5] | string |
[title_h6] | string |
[finish_text] | string |
[title_description] | string |
[submit_button] | string |
[submit_button_processing] | string |
Preview
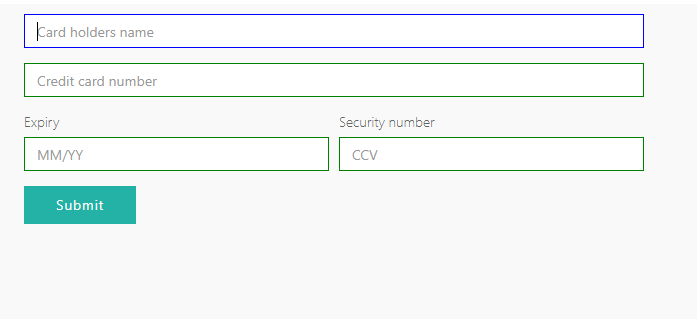
setFormValues
Description
Method to set predefined values for the form fields inside the widget.
widget.setFormValues({
email: '[email protected]',
card_name: 'Houston'
});
Configurable Parameters
Parameter | Type |
---|---|
card_name | string |
expire_month | string |
expire_year | string |
card_ccv | string |
first_name | string |
last_name | string |
string | |
phone | string |
phone2 | string |
address_line1 | string |
address_line2 | string |
address_state | string |
address_city | string |
address_postcode | string |
address_company | string |
Preview
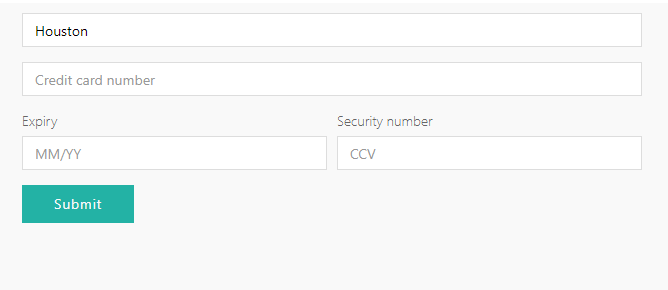
setFormLables
Description
Method to set custom form field labels.
widget.setFormLabels({
card_name: 'Card Holder Name ABC'
})
Configurable Parameters
Parameter | Type |
---|---|
card_name | string |
expire_month | string |
expire_year | string |
card_ccv | string |
first_name | string |
last_name | string |
string | |
phone | string |
phone2 | string |
address_line1 | string |
address_line2 | string |
address_state | string |
address_city | string |
address_postcode | string |
address_company | string |
Preview
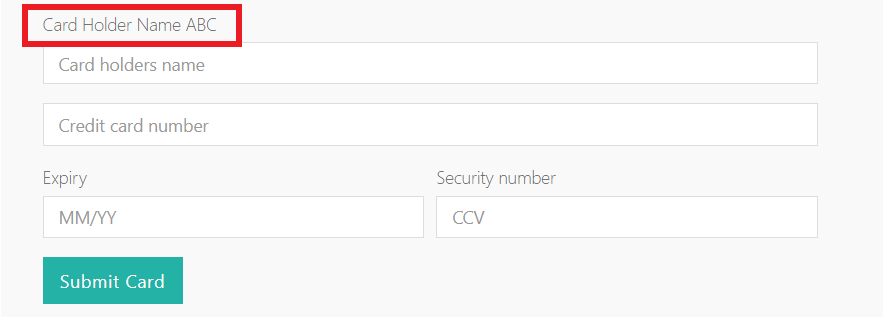
setFormPlaceholders
Description
Method to set custom form field labels.
widget.setFormPlaceholders({
card_name: 'Input your card holder name...',
email: 'Input your email, like [email protected]'
})
Configurable Parameters
Parameter | Type |
---|---|
card_name | string |
expire_month | string |
expire_year | string |
card_ccv | string |
first_name | string |
last_name | string |
string | |
phone | string |
phone2 | string |
address_line1 | string |
address_line2 | string |
address_state | string |
address_city | string |
address_postcode | string |
address_company | string |
Preview
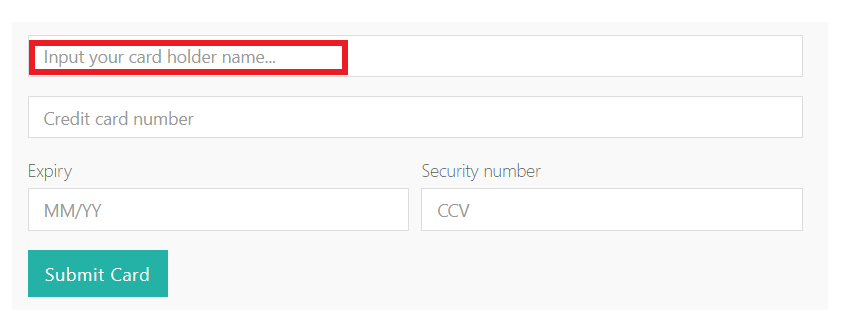
setHiddenElements
Description
Using this method, you can set hidden elements for the widget.
widget.setHiddenElements(['submit_button']);
Configurable Parameters
Parameters | Type |
---|---|
SUBMIT_BUTTON | string |
Preview
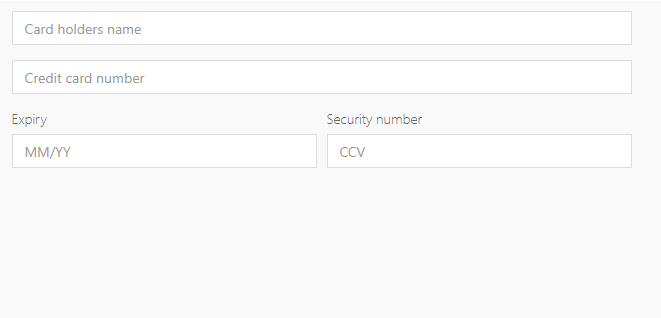
setRefId
Description
Method to set a custom reference when using the widget.
widget.setRefId('abc-1234567');
Configurable Parameters
Parameters | Type |
---|---|
id | string |
Preview
{
"event": "finish",
"purpose": "payment_source",
"message_source": "widget.paydock",
"ref_id": "abc-1234567",
"widget_id": "7ff7ff74-dedb-236d-0383-4d7afc8ad462",
"payment_source": "27f2efcf-df0c-4d3e-b094-17d272c4ba97"
}
setSupportedCardIcons
Description
Sets icons of supported card types.
widget.setSupportedCardIcons(['mastercard', 'visa', 'amex']);
Configurable Parameters
Parameters | Type | default |
---|---|---|
AMEX | string | "amex" |
AUSBC | string | "ausbc" |
DINERS | string | "diners" |
DISCOVER | string | "discover" |
JAPCB | string | "japcb" |
LASER | string | "laser" |
MASTERCARD | string | "mastercard" |
SOLO | string | "solo" |
VISA | string | "visa" |
VISA_WHITE | string | "visa_white" |
Preview
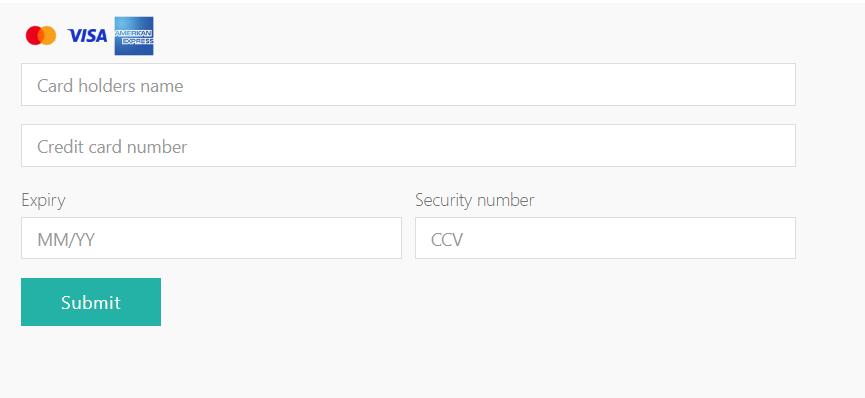
validateCardNumberInput
Description
Validation extension will only enforce the supported cards listed prior and everything else will get a not supported prompt
widget.setSupportedCardIcons(['mastercard', 'visa'], validateCardNumberInput = true);
setWebHookDestination
Description
Destination where customer will receive all successful responses. Response will contain “data” object with “payment_source” or other parameters, in depending on 'purpose'
widget.setWebHookDestination('http://google.com');
Configurable Parameters
Parameters | Type | Description |
---|---|---|
url | string | Your endpoint for POST request |
setSuccessRedirectUrl
Description
URL to which the Customer will be redirected to after the success finish
widget.setSuccessRedirectUrl('google.com/search?q=success');
Configurable Parameters
Parameters | Type |
---|---|
url | string |
setErrorRedirectUrl
Description
URL to which the Customer will be redirected to if an error is triggered in the process of operation
widget.setErrorRedirectUrl('google.com/search?q=error');
Configurable Parameters
Parameters | Type |
---|---|
url | string |
setFormFields
Description
Set list with widget form field, which will be shown in form. Also you can set the required validation for these fields
widget.setFormFields(['phone', 'email', 'first_name*']);
Configurable Parameters
Parameters | Type | Description |
---|---|---|
fields | [ 'Array' ]. | name of fields which can be shown in a widget. If after a name of a field, you put “*”, this field will be required on client-side. (For validation, you can specify any fields, even those that are shown by default: card_number, expiration, etc... ) |
load
Description
The final method to beginning, the load process of widget to html
on
Description
htmlWidget.on(eventName, [cb]) ⇒ Promise.<(IEventData|IEventMetaData|IEventFinishData|IFormValidation)> | void
Listen to events of widget.
widget.on('form_submit', function (data) {
console.log(data);
});
// or
widget.on('form_submit').then(function (data) {
console.log(data);
});
Configurable Parameters
Parameters | Type | Description |
---|---|---|
eventName | string | Available events found here: events |
trigger
Description
This callback will be called for every specified trigger
Configurable Parameters
Parameters | Type | Description |
---|---|---|
triggers | triggerName | submit_form, tab |
data | ITriggerData | Data which will be sent to the widget |
getValidationState
Description
This method will check the validation state of the form
Returns IFormValidation response
widget.getValidationState();
isValidForm
Description
This method will check if the form is valid
Returns Boolean - Form is valid
widget.isValidForm();
isInvalidForm
Description
Using this method you can check if a specific form field is invalid
Returns Boolean - Field is invalid
widget.isInvalidField(field);
Configurable Parameters
Parameters | Type | Description |
---|---|---|
field | string | field name |
isInvalidFieldByValidator
Description
Using this method you can check if a specific field is invalid
Returns Boolean - Field is invalid
widget.isInvalidFieldByValidator(field, validator);
Configurable Parameters
Parameters | Type | Description |
---|---|---|
field | string | field name |
validator | string | Validator name: Available values: required, cardNumberValidator, expireDateValidation |
hide
Description
Using this method you can hide the widget after load
widget.hide([saveSize = False]);
Configurable Parameters
Parameters | Type | Description |
---|---|---|
saveSize | Boolean | Using this param you can save the iFrame's size. Default = false |
show
Description
This method will show the widget after using hide
widget.show()
reload
Description
This method will reload the widget
widget.reload()
hideElements
Description
Use this method to hide any elements inside the widget
widget.hideElements(['submit_button', 'email']);
Configurable Parameters
Parameters | Type | Description |
---|---|---|
elements | array | List of elements which will be hidden |
interceptSubmitForm
Description
Widget will intercept submit of your form for processing widget
Process: click by submit button in your form --> submit widget ---> submit your form
Kind: instance method of HtmlWidget
Note: submit button in widget will be hidden.
<form id="myForm">
<button type="submit">Submit</button>
</form>
<!--
-->
<script>
widget.interceptSubmitForm('#myForm');
</script>
showElements
Description
Use this method to show elements inside the widget
widget.showElements(['submit_button', 'email']);
Configurable Parameters
Parameters | Type | Description |
---|---|---|
elements | array | List of elements which will be shown |
updateFormValues
Description
Method for updating values of the form fields within the widget
widget.updateFormValues({
email: '[email protected]',
card_name: 'Houston'
});
Configurable Parameters
Parameters | Type | Description |
---|---|---|
fieldValues | IFormValues | List of fields and values to be updated |
onFinishInsert
Description
After the finish event of the widget, data (dataType) will be inserted into the input (selector)
widget.onFinishInsert(selector, dataType);
Configurable Parameters
Parameters | Type | Description |
---|---|---|
selector | string | css selector |
dataType | string | data type of IEventData Object |
useAutoResize
Description
Use this method to resize the iFrame according to content height
widget.useAutoResize();
usePhoneCountryMask
Description
Method to set a country code mask for the phone input
widget.usePhoneCountryMask([options]);
Configurable Parameters
Parameters | Type | Description |
---|---|---|
options | Object | Object defining the configurable options for the phone mask |
option.default_country | string | Set a default country for the mask |
options.preferred_countries | array | Set list of preferred countries for the top of the select box |
options.only_countries | array | Set list of countries to show in the select box. |
Events
List of available event names
Event name | type | default | Response |
---|---|---|---|
AFTER_LOAD | string | "afterLoad" | Returns IEventData event object |
SUBMIT | string | "submit" | Returns IEventData event object |
FINISH | string | "finish" | Returns IEventFinishData event object |
VALIDATION | string | "validation" | Returns IFormValidation event object |
VALIDATION_ERROR | string | "validationError" | Returns IFormValidation event object |
SYSTEM_ERROR | string | "systemError" | Returns IEventData event object |
META_CHANGE | string | "metaChange" | Returns IEventMetaData event object |
RESIZE | string | "resize" | Returns IEventData event object |
Interfaces
IEventData
Parameter | Type | Description |
---|---|---|
event | string | Event name |
purpose | string | system variable. Purpose of the event |
message_source | string | system variable. Event source |
ref_id | string | custom value for identifying the result of the processed operation |
IEventMetaData
Parameter | Type | Description |
---|---|---|
event | string | Event name |
purpose | string | system variable. Purpose of the event |
message_source | string | system variable. Event source |
ref_id | string | custom value for indentifying the result of the processed operation |
configuration_token | string | Token received from our API with widget data |
type | string | Payment type, 'card' or 'bank account' |
gateway_type | string | Gateway type |
card_number_last4 | string | Last 4 digits of the card |
card_scheme | string | Card Scheme |
card_number_length | string | Card number length |
IEventFinishData
Parameter | Type | Description |
---|---|---|
event | string | Event name |
purpose | string | system variable. Purpose of the event |
message_source | string | system variable. Event source |
ref_id | string | custom value for identifying the result of the processed operation |
payment_source | string | One time token returned from PowerBoard |
IFormValidation
Parameter | Type | Description |
---|---|---|
event | string | Event name |
purpose | string | system variable. Purpose of the event |
message_source | string | system variable. Event source |
ref_id | string | custom value for identifying the result of the processed operation |
form_valid | boolean | Boolean identifying if the form is valid |
invalid_fields | array | invalid form fields |
invalid_showed_fields | array | list of fields on which the error is already displayed |
validators | array | list of validators with fields |